Java集合根据某 单个/多个 字段的值是否相同,判重、去重
Java-Mybatis-批量查询、list转map
集合框架图
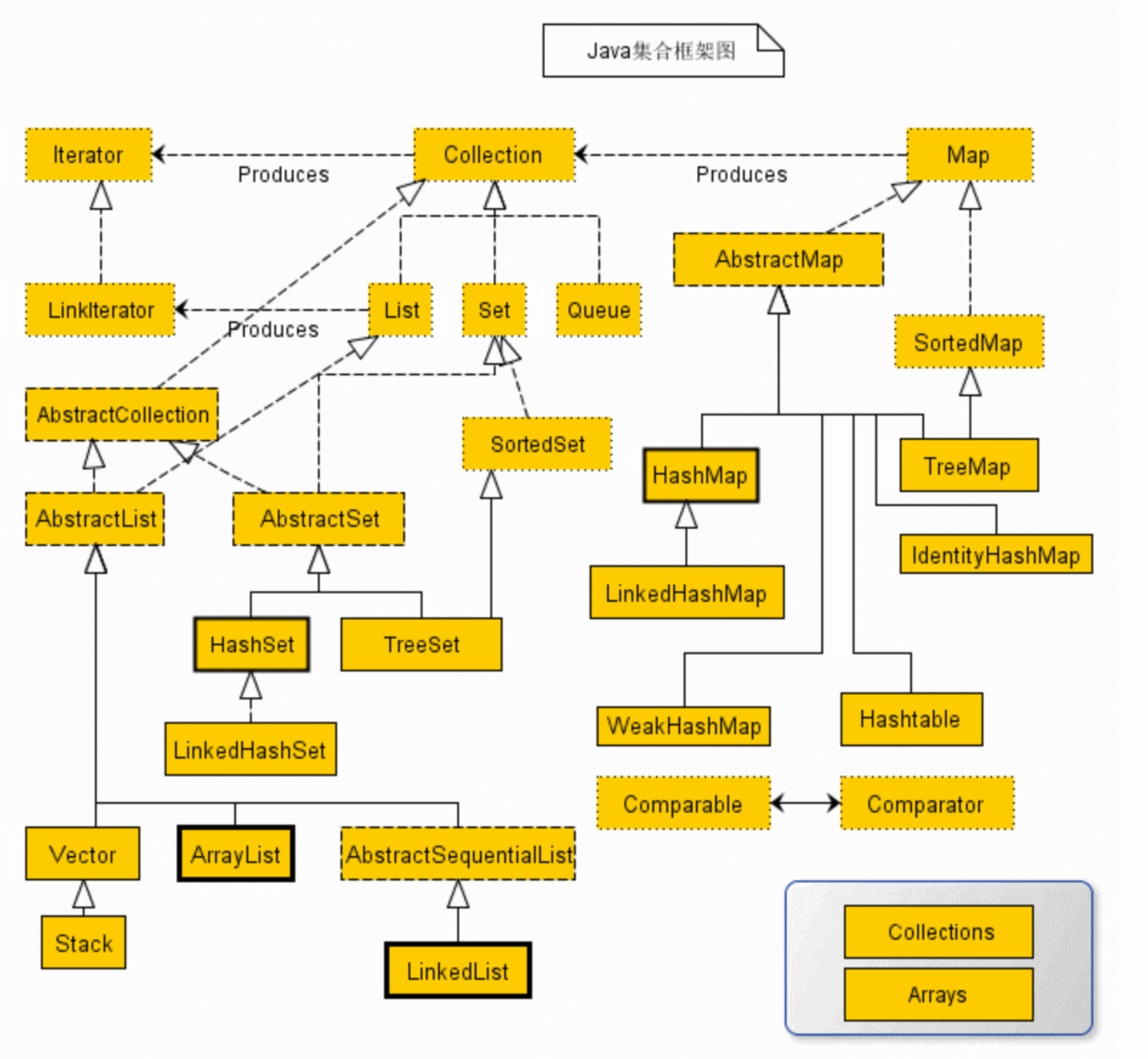
list集合常用排序方法
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
// comparingInt/comparingLong/comparingDouble
// 根据某字段倒排
entityList = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(s -> s.getKey() != null)
.sorted(comparing(s -> s.getKey()).reversed())
.collect(Collectors.toList());
// 倒叙并取第一个对象
Optional<Entity> first = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(s -> s.getKey() != null)
.sorted(Comparator.comparing(s -> s.getKey()).reversed())
.findFirst();
// null放最后
entityList = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(s -> s.getKey() != null)
.sorted(comparing(s -> s.getKey())
.thenComparing(User::getAge, Comparator.nullsLast(Comparator.naturalOrder())));
|
List集合自定义排序规则,获取优先级最高的一个
枚举类型 自定义优先级
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
Optional<String> min = Optional.ofNullable(stringList).orElse(new ArrayList<>())
.stream()
.min(Comparator.comparing(s -> {
if (ROLE_FLEET.getCode().equals(s)) {
return 1;
}
if (ROLE_DRIVER.getCode().equals(s)) {
return 2;
}
if (ROLE_VISITOR.getCode().equals(s)) {
return 3;
}
return 99;
}));
min.ifPresent(s -> roles.add(BrpRole.builder().code(s).build()));
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
Optional.ofNullable(brpRoles).orElse(new ArrayList<>())
.stream().map(BrpRole::getCode)
.filter(s -> !ROLE_STATION_OPERATIONS.getCode().equals(s))
.min(Comparator.comparing(s -> {
if (ROLE_FLEET.getCode().equals(s)) {
return 1;
}
if (ROLE_DRIVER.getCode().equals(s)) {
return 2;
}
if (ROLE_VISITOR.getCode().equals(s)) {
return 3;
}
return 99;
}))
.ifPresentOrElse(s -> infoVo.setUserType(s),
() -> infoVo.setUserType(ROLE_VISITOR.getCode()));
|
返回较大的时间
1
2
3
4
5
6
7
8
9
10
11
12
|
Map<String, Date> keyDateValueMap = Optional.ofNullable(list).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.filter(s -> s.getDateValue() != null)
.collect(Collectors.toMap(
s -> s.getKey(),
s -> s.getDateValue(),
(s1, s2) -> {
LocalDateTime time1 = LocalDateTime.ofInstant(s1.toInstant(), ZoneId.systemDefault());
LocalDateTime time2 = LocalDateTime.ofInstant(s2.toInstant(), ZoneId.systemDefault());
return time1.isAfter(time2) ? s1 : s2;
}
));
|
Java集合转字符串、并写到文件、StringBuilder拼接字符串
1
2
3
4
5
6
|
<!-- https://mvnrepository.com/artifact/com.google.guava/guava -->
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>33.2.1-jre</version>
</dependency>
|
map转string
1
2
3
|
Map<Integer, String> map = Map.of(1, "a", 2, "b", 3, "c");
// 1=a,2=b,3=c
String string = Joiner.on(",").withKeyValueSeparator("=").join(map);
|
StringBuilder拼接字符串
1
2
3
4
|
StringBuilder stringBuilder = new StringBuilder("hello、");
// hello、foo|bar|baz
StringBuilder stringBuilder1 = Joiner.on("|").skipNulls()
.appendTo(stringBuilder, "foo", "bar", null, "baz");
|
连接List元素并写到文件流
1
2
3
4
5
6
7
8
9
|
try (FileWriter fileWriter = new FileWriter(类名.class.getResource("/").getPath() + "test" + File.separator + "tmp.txt", false)) {
List<Date> dateList = Lists.newArrayList(new Date(), null, new Date());
Joiner joiner2 = Joiner.on("#").useForNull("no string");
// 是否覆盖取决于fileWriter的打开方式,默认是覆盖,若有true,则是追加
joiner2.appendTo(fileWriter, dateList);
} catch (IOException e) {
System.out.println(e.getMessage());
}
|
list转string
1
2
3
|
List<String> list = Lists.newArrayList("a", "b");
// a,b 如果集合初始化并且没数据,则直接为空字符串;guava使用快速失败操作,在编写时告警未初始化集合
String string = Joiner.on(",").join(list);
|
对象List集合,根据对象某个集合字段拆分成两条数据
1
2
3
4
5
6
7
8
|
@Data
public class AppUserDTO {
private String userId;
private String appUserType;
private List<String> appUserTypeList;
// 构造函数、getter和setter省略
}
|
1
2
|
// 调用
appUserDTOList = splitAppUserDTOs(appUserDTOList);
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
import java.util.ArrayList;
import java.util.List;
public class AppUserSplitter {
public static List<AppUserDTO> splitAppUserDTOs(List<AppUserDTO> appUserDTOList) {
List<AppUserDTO> result = new ArrayList<>();
for (AppUserDTO original : appUserDTOList) {
if (original.getAppUserTypeList().size() > 1) {
// 对于每个原始对象,如果appUserTypeList包含多个元素,则拆分
for (String type : original.getAppUserTypeList()) {
AppUserDTO newDTO = new AppUserDTO();
// 复制除appUserTypeList外的所有字段
newDTO.setUserId(original.getUserId());
// 设置拆分后的appUserType
newDTO.setAppUserType(type);
newDTO.setAppUserName(AppRole.getByCode(type).getDesc());
// 将新的DTO添加到结果列表中
result.add(newDTO);
}
} else if (original.getAppUserTypeList().size() == 1) {
// 如果appUserTypeList只有一条,直接添加到结果列表中
original.setAppUserType(original.getAppUserTypeList().get(0));
if (!ObjectUtils.isEmpty(original.getAppUserTypeNameList())) {
original.setAppUserName(original.getAppUserTypeNameList().get(0));
}
result.add(original);
} else {
result.add(original);
}
}
return result;
}
}
|
条件查询-条件框可多选情况、多条件查询
推荐文章
字符串转List集合
1
2
3
4
5
6
|
<!-- https://mvnrepository.com/artifact/com.google.guava/guava -->
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>33.2.1-jre</version>
</dependency>
|
实现方式1
1
|
@Parameter(description = "用户类型") @RequestParam(required = false, name = "userType") List<String> userTypeList,
|
实现方式2
1
2
|
@Parameter(description = "交易类型 多个用英文逗号拼接 如a,b,c")
@RequestParam(required = false) String transactionType,
|
1
2
3
|
List<String> transactionTypeList = StringUtils.isBlank(transactionType) || "all".equals(transactionType) ? Collections.emptyList() :
Splitter.on(",").omitEmptyStrings().trimResults()
.splitToList(transactionType);
|
mapList中两个map合并
以下演示将key=key2的map中的total加到key=key1的map中total上,并移除key=key1的map
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
public static void main(String[] args) {
List<Map<String, Object>> list = new ArrayList<>();
Map<String, Object> map1 = new HashMap<>();
map1.put("key", "key1");
map1.put("total", 1);
list.add(map1);
Map<String, Object> map2 = new HashMap<>();
map2.put("key", "key2");
map2.put("total", 2);
list.add(map2);
if (!ObjectUtils.isEmpty(list)) {
Integer total1 = 0, total2 = 0;
for (Map<String, Object> map : list) {
if ("key1".equals(map.get("key"))) {
total1 = (Integer) map.get("total");
}
if ("key2".equals(map.get("key"))) {
total2 = (Integer) map.get("total");
}
}
for (Map<String, Object> map : list) {
if ("key1".equals(map.get("key"))) {
map.put("total", total1 + total2);
}
}
list.removeIf(map -> "key2".equals(map.get("key")));
}
}
|
list转map
相关文章:Mybatis批量查询
单keyEntityListMap
1
2
3
4
5
|
Map<String, List<Entity>> keyListMap = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.collect(groupingBy(s -> s.getKey()));
List<Entity> enetityList = Optional.of(keyListMap).map(s -> s.get(key)).orElse(new ArrayList<>());
|
map1中拿到value, 作为key,去map2中取value
1
2
3
4
5
6
7
|
Map<Long, String> key1Key2Map = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.collect(Collectors.toMap(s -> s.getKey1(), s -> s.getKey2(), (s1, s2) -> s1));
String key2 = Optional.of(key1Key2Map).map(s -> s.get(key1)).orElse("");
List<String> enetityList = Optional.of(key2EntityListMap).map(s -> s.get(key2)).orElse("");
|
单keyEntityMap
1
2
3
4
5
6
|
Map<String, Entity> keyEntityMap = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.collect(Collectors.toMap(s -> s.getKey(), Function.identity(), (s1, s2) -> s1));
Optional<Entity> entityOptional = Optional.of(keyEntityMap).map(s -> s.get(key));
String value = entityOptional.map(s -> s.getValue()).orElse("");
|
单keyValueMap
1
2
3
4
5
6
|
Map<String, String> keyValueMap = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.filter(s -> s.getValue() != null)
.collect(Collectors.toMap(s -> s.getKey(), s -> s.getValue(), (s1, s2) -> s1));
String value = Optional.of(keyValueMap).map(s -> s.get(key)).orElse("");
|
value:返回较大的时间
1
2
3
4
5
6
7
8
9
10
11
12
|
Map<String, Date> keyDateValueMap = Optional.ofNullable(list).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.filter(s -> s.getDateValue() != null)
.collect(Collectors.toMap(
s -> s.getKey(),
s -> s.getDateValue(),
(s1, s2) -> {
LocalDateTime time1 = LocalDateTime.ofInstant(s1.toInstant(), ZoneId.systemDefault());
LocalDateTime time2 = LocalDateTime.ofInstant(s2.toInstant(), ZoneId.systemDefault());
return time1.isAfter(time2) ? s1 : s2;
}
));
|
双key依赖
1
2
3
4
|
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
</dependency>
|
双key-pairListMap
1
2
3
4
5
|
Map<Pair<Long, String>, List<Entity>> key1key2ListMap = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.collect(groupingBy(s -> Pair.of(s.getKey1(), s.getKey2())));
List<Demo> entityList = Optional.of(key1key2ListMap).map(s -> s.get(Pair.of(key1, key2))).orElse(new ArrayList<>());
|
双key-pairEntityMap
1
2
3
4
5
6
|
Map<Pair<Long, String>, Demo> key1key2EntityMap = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.collect(Collectors.toMap(s -> Pair.of(s.getKey1(), s.getKey2()), Function.identity(), (s1, s2) -> s1));
Optional<Entity> entityOptional = Optional.of(key1key2EntityMap).map(s -> s.get(Pair.of(key1, key2)));
String value = entityOptional.map(s -> s.getValue()).orElse("");
|
双key-pairValueMap
1
2
3
4
5
6
|
Map<Pair<Long, String>, String> key1key2ValueMap = Optional.ofNullable(entityList).orElse(new ArrayList<>())
.stream().filter(Objects::nonNull)
.filter(s -> s.getValue() != null)
.collect(Collectors.toMap(s -> Pair.of(s.getKey1(), s.getKey2()), s -> s.getValue(), (s1, s2) -> s1));
String value = Optional.of(key1key2ValueMap).map(s -> s.get(Pair.of(key1, key2))).orElse("");
|